People around the globe use Facebook, Instagram, Pinterest, Twitter and many other popular applications every day. They’re all used for different purposes like staying in touch with friends, creating blogs, posting pictures, or discovering ideas. However, one thing that users of these apps have in common is that every single day they scroll through the apps’ activity feeds searching for new updates. A global activity feed is like a forum where users can post photos or make comments, as well as keep track of recent news or friends and family’s lives. In this article, we’ll be focusing on how to build one using React Native and Node.js.
Before we start
Adding a global activity feed to a mobile application sounds difficult, but, luckily for us, there are third-party libraries to help us, which is one of the things that we like most about React Native.
Today, we’ll use react-native-activity-feed and also, as the documentation states, we’ll need to include the react-native-image-crop-picker if we are using native packages or expo-image-picker if we are using Expo.
To install all of these libraries, run the following scripts on your terminal from your React Native root project folder.
If you are using Expo:
- expo install expo-activity-feed expo-permissions expo-image-picker
If you are using native packages:
- npm install react-native-activity-feed OR yarn add react-native-activity-feed
- npm install react-native-image-crop-picker OR yarn add react-native-image-crop-picker
Remember that, if you are using Xcode, every time you add new libraries to your project you have to run npx pod-install to install pods dependencies.
Important note: to use react-native-activity-feed you need to have a GetStream account (we’ll look at this in the following steps). That said, GetStream provides paid plan options to use its resources; you can still use the Activity Feed package for free for development purposes, but only for a limited time.
Let’s jump into the code of a Global Activity Feed using React Native
First of all, you need to create a React Native project.
If you don’t know how to create your app, just run the following commands on your terminal:
Now that you’ve created the application, let’s get to coding!
Important note: this example was made using React Native 0.66.
First steps
First, we need to create an account on https://getstream.io/accounts/signup/
After signing up, we’ll navigate to our dashboard where we’ll see that an application has already been created for us. This app includes an App ID, a Key and a Secret Key. We will use those values later.
By clicking on “PRODUCTION APP”, we can access our ‘Feeds’, access keys and other information. Note that we can create new feeds in this section.
Express backend client
It is very important to know that we can’t use our Secret Key inside a desktop/mobile/web environment, so we need a backend client.
Therefore, in a separate folder from the one containing the mobile project, we will run npm init in our console and provide a project name (e.g.: backend-server).
Inside this folder we need to install the two libraries using the following commands:
- npm install express –save
- npm install getstream
Link: Backend.js
This Express server is very minimalist and its main purpose is to allow us to create a user token for the frontend application. Keep in mind that we are always creating and or getting and subscribing to the feed using a user with id ‘tester’. If we want to add more users, we can change this or send the param inside a query param.
With the server running, now we have to fetch a request to, in this case, http://localhost:3000 from the mobile application.
Mobile application
Link: App.js
In this example, we have an activity indicator running while we get the user access token to start our StreamApp. The only two values we have here are the STREAM_API_KEY and STREAM_APP_ID; the token is the one that we got from the backend.
GetStream provides many components to use and customize. The most important one to establish the connection to our feed is StreamApp, which includes the API key, the app ID and a private token. We also need to send our user ID to see our user information in the post we create.
We can customize our feed however we want. We can also copy/paste the GetStream components to our code and make some modifications. In this example, we’ll customize the UserBar component for every Activity, and we’ll also add a LikeButton easily and in just a few lines as you can see in the link above!
Finally, we will see how the notify prop on the FlatFeed component shows that, every time there is a new post available, we will be notified and can tap on the notification area to see the new posts. If we prefer swiping down to update the feed, we can set the notify prop to false.
Global Activity Feed preview
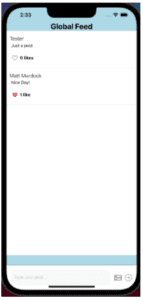
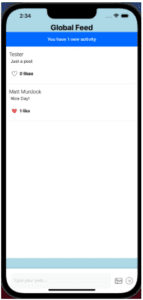
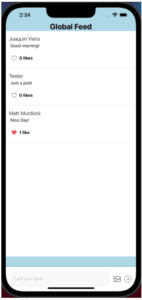
Final thoughts on building a Global Activity Feed using React Native
As we’ve seen, adding an activity feed to our React Native application can be done with just a few lines of code and it is not as hard as it might seem.
The best advantages of this library are that it is easy to use, it is easy to adapt and customize to our designs, and it provides many other features to use in our development projects.